React Hooks | Most Essential Hooks
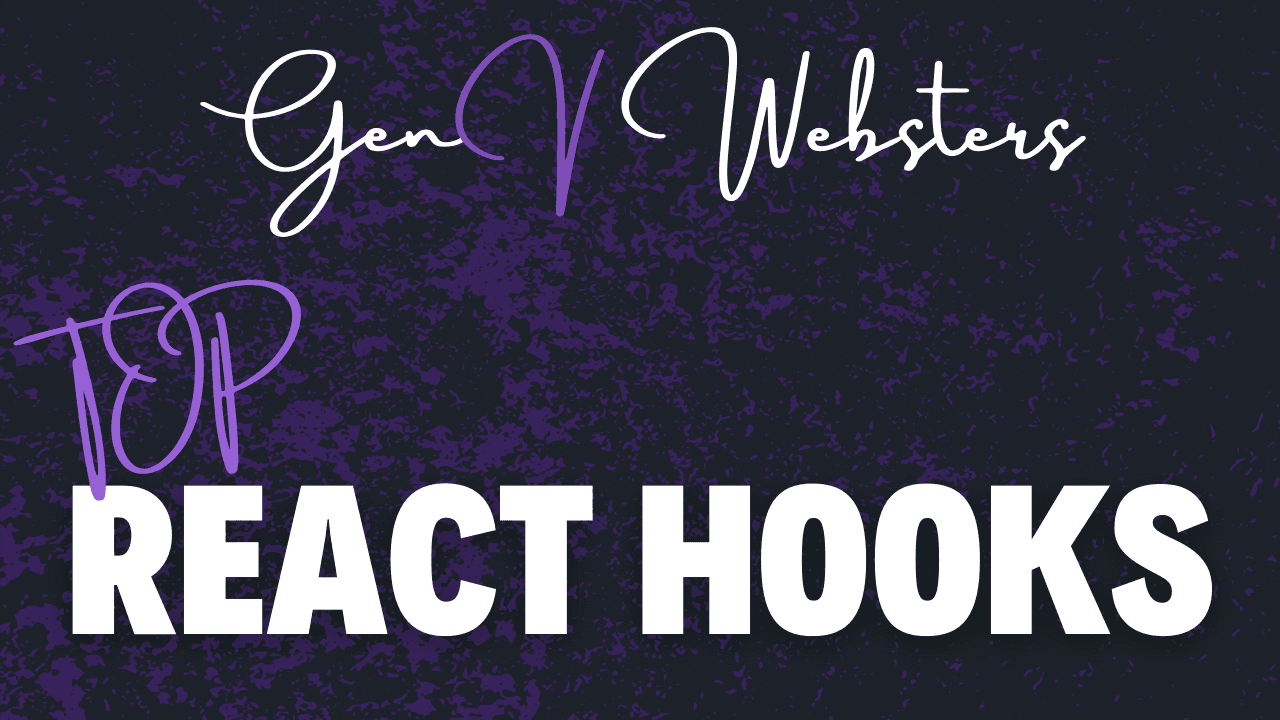
Introduction
Discover how React Hooks revolutionize state and lifecycle management in functional components. Learn essential Hooks like useState and useEffect for your projects.

1. useState Hook
The useState
Hook manages local component state. It returns an array with the current state and a function to update it.
Syntax:
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
}
Use Case: Managing local component state like form inputs, toggle buttons, counters, etc.

2. useEffect Hook
The useEffect
Hook handles side effects in functional components, such as fetching data, setting up event listeners, and subscriptions.
Syntax:
import { useState, useEffect } from 'react';
function DataFetcher() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>;
}
Use Case: Fetching data, DOM manipulations, and integrating third-party libraries.

3. useContext Hook
The useContext
Hook allows access to global state without prop drilling.
Syntax:
import { createContext, useContext } from 'react';
const ThemeContext = createContext('light');
function ThemedComponent() {
const theme = useContext(ThemeContext);
return <div className={theme}>This is a {theme}-themed component.</div>;
}
Use Case: Sharing global state like themes, user authentication, or language settings.

4. useRef Hook
The useRef
Hook creates references to DOM elements or stores values that persist without re-renders.
Syntax:
import { useRef } from 'react';
function FocusInput() {
const inputRef = useRef(null);
const focusInput = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={focusInput}>Focus Input</button>
</div>
);
}
Use Case: Managing focus, accessing DOM elements, and persisting values across renders.

5. useMemo Hook
The useMemo
Hook improves performance by memoizing calculations, preventing unnecessary re-renders.
Syntax:
import { useState, useMemo } from 'react';
function ExpensiveCalculationComponent({ num }) {
const result = useMemo(() => {
return num * 2; // Simulate an expensive computation
}, [num]);
return <p>Result: {result}</p>;
}
Use Case: Avoiding costly re-calculations in performance-sensitive components.

6. useCallback Hook
The useCallback
Hook memoizes functions, preventing unnecessary re-renders of child components.
Syntax:
import { useCallback } from 'react';
function Button({ handleClick }) {
return <button onClick={handleClick}>Click Me</button>;
}
function ParentComponent() {
const handleClick = useCallback(() => {
console.log('Button clicked!');
}, []);
return <Button handleClick={handleClick} />;
}
Use Case: Optimizing event handlers in child components.

7. useReducer Hook
The useReducer
Hook is an alternative to useState
, ideal for managing complex state logic.
Syntax:
import { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
Use Case: Handling intricate state logic, including forms or global state management.

Conclusion
React Hooks simplify state management and make functional components more powerful. Mastering essential Hooks—useState
, useEffect
, useContext
, useRef
, useMemo
, useCallback
, and useReducer
—will help you build efficient and maintainable applications.

By mastering these Hooks, you can write cleaner and more optimized React applications. If you’re looking for a React Components, bookmark this guide for quick reference!