React Components
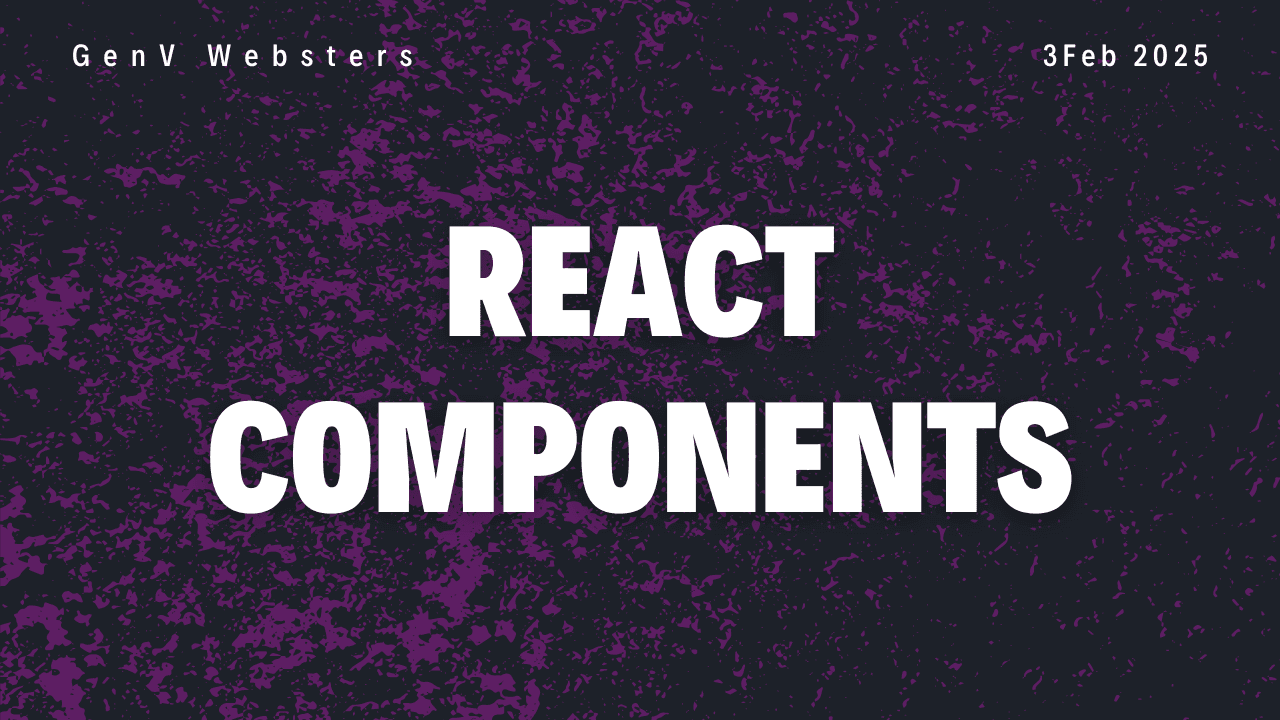
The Ultimate Guide to React Components: Everything You Need to Know
Introduction to React Components
React has revolutionized the way we build web applications by introducing the concept of components. A React component is a reusable piece of UI that encapsulates logic and rendering. Whether you’re a beginner or an advanced developer, understanding React components is essential for building scalable and maintainable applications.
In this guide, we will explore different types of React JS components, their usage, and best practices. By the end of this blog, you’ll have a solid understanding of how to leverage components in React efficiently.
Suggested Image: A diagram showing the structure of a React component with JSX, props, and state.
1. What Are React Components?
A React component is a JavaScript function or class that returns a piece of UI. Components allow developers to break down complex UIs into smaller, manageable parts.
Types of React Components:
- Functional Components – These are simple JavaScript functions that accept props and return JSX.
- Class Components – These are ES6 classes that extend
React.Component
and have lifecycle methods.
Example of a Functional Component:
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
Functional components are preferred in modern React development because they are simpler and support hooks.

2. Understanding Props and State in React Components
Props (Properties) and State are two key concepts in React components that determine how data is managed and displayed.
Props:
Props are read-only attributes passed from a parent component to a child component.
function Greeting(props) {
return <h1>Good Morning, {props.name}!</h1>;
}
State:
State is used to manage dynamic data within a component.
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Suggested Image: A flowchart showing how props and state interact within a React component.
3. Types of React Components: Stateless vs. Stateful
React components can be categorized based on their ability to manage state.
Stateless Components:
- Do not manage state.
- Only render UI based on props.
- Ideal for presentational components.
Example:
function DisplayMessage({ message }) {
return <p>{message}</p>;
}
Stateful Components:
- Maintain their own state.
- Handle logic and interactivity.
Example:
class Timer extends React.Component {
constructor(props) {
super(props);
this.state = { seconds: 0 };
}
componentDidMount() {
this.interval = setInterval(() => {
this.setState({ seconds: this.state.seconds + 1 });
}, 1000);
}
componentWillUnmount() {
clearInterval(this.interval);
}
render() {
return <p>Timer: {this.state.seconds} seconds</p>;
}
}
Suggested Image: Infographic explaining the lifecycle of stateful components.
4. Lifecycle Methods and Hooks in React Components
Lifecycle Methods (Class Components):
Class components have built-in lifecycle methods such as:
componentDidMount()
: Runs after the component is inserted into the DOM.componentDidUpdate()
: Executes when the component updates.componentWillUnmount()
: Runs before the component is removed from the DOM.
Hooks (Functional Components):
With React hooks, functional components can manage state and side effects:
- useState(): Manages local state.
- useEffect(): Handles side effects.
Example:
import { useEffect, useState } from 'react';
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(seconds + 1);
}, 1000);
return () => clearInterval(interval);
}, [seconds]);
return <p>Timer: {seconds} seconds</p>;
}
Suggested Image: Comparison table between class-based lifecycle methods and hooks.
5. Best Practices for Using React Components
To ensure your React components are efficient and maintainable, follow these best practices:
1. Keep Components Small and Focused
Each component should have a single responsibility to make code easier to maintain.
2. Use PropTypes for Type Safety
import PropTypes from 'prop-types';
function Button({ label }) {
return <button>{label}</button>;
}
Button.propTypes = {
label: PropTypes.string.isRequired,
};
3. Optimize Performance with Memoization
Use React.memo()
to prevent unnecessary re-renders.
const MemoizedComponent = React.memo(MyComponent);
4. Use Key Props in Lists
When rendering lists, always include a unique key
prop.
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
Suggested Image: Illustration showing performance optimization in React.
Conclusion
Mastering React components is crucial for efficient and scalable web development. By understanding different types of components in React, their lifecycle, and best practices, you can build highly interactive applications with ease.
Whether you’re just starting or looking to improve your React JS components, this guide provides the foundation needed to write clean, reusable, and efficient code.